Caching in Next.js: A Detailed Overview
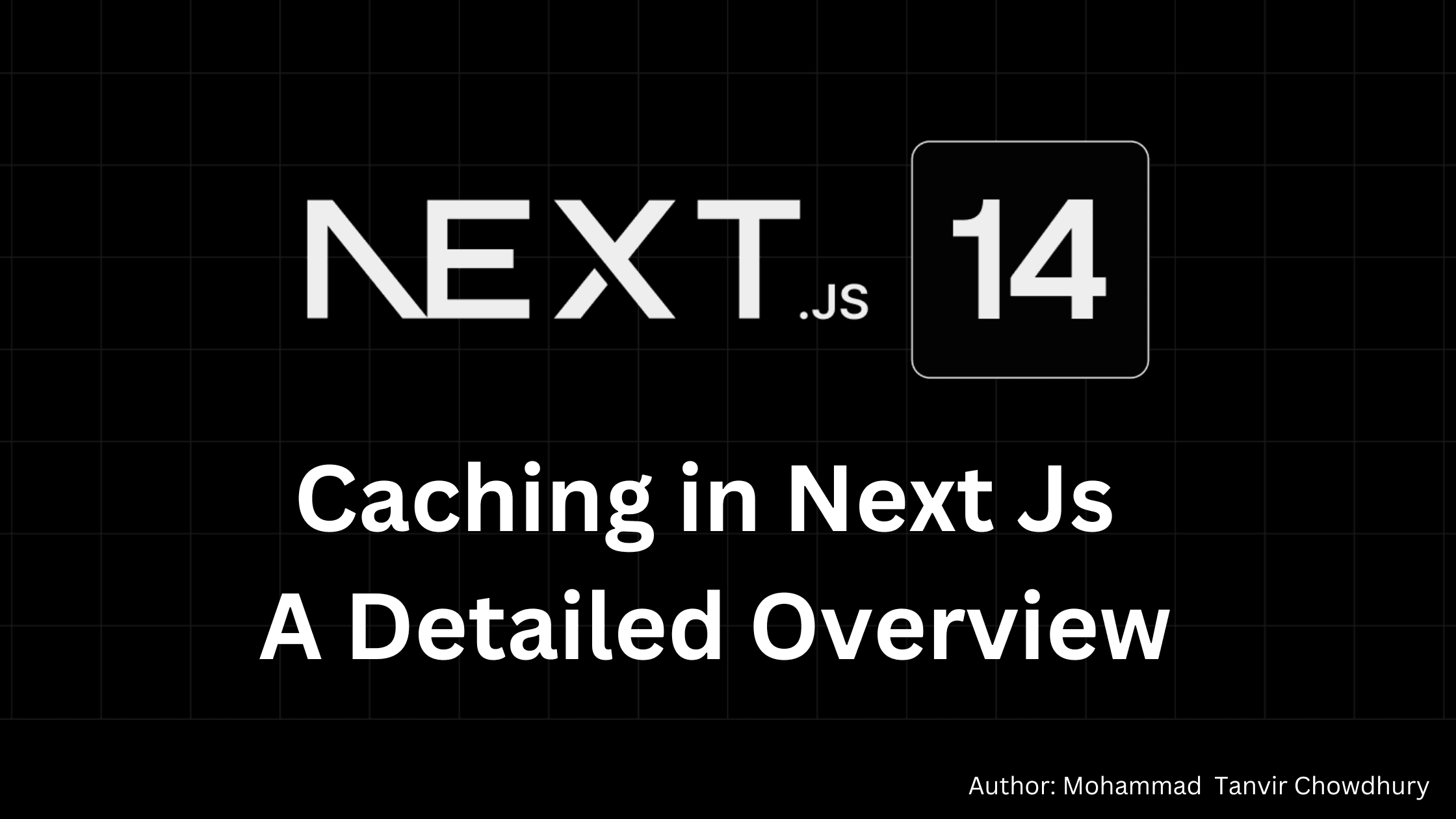
Next.js provides robust caching mechanisms that help improve the performance and efficiency of web applications. Understanding these mechanisms can guide you in building faster and more responsive applications. Below are the four primary caching strategies employed by Next.js:
Overview of Caching Mechanisms
Next.js 14 employs four primary caching mechanisms:
Request Memoization
Data Cache
Full Route Cache
Router Cache
Each caching type plays a role in how your application fetches and serves data.
1. Request Memoization
- Where: Server
- What: Caches similar GET requests that share the same URL and options. This means that if the same data is requested multiple times in a single page request, Next.js will return the cached result rather than making a new network request.
- Duration: The cache lasts for the duration of the page request. This is particularly useful for minimizing redundant fetches in components that may request the same data multiple times during a single rendering.
- Benefit: Reduces redundant fetches in components, which enhances performance and decreases load times. It ensures that your application is efficient, especially when fetching data that does not change frequently.
- Opt-out: You can use AbortController to skip caching for specific requests. This is helpful when you need to ensure that a particular request is always fresh.
2. Data Cache
- Where: Server
- What: This cache stores data retrieved from routes or single fetch requests. It is especially useful for storing data fetched from APIs or databases.
- Duration: The Data Cache is indefinite, meaning it survives server restarts and deployments. This makes it a powerful tool for managing persistent data.
- Revalidation: You have several options for revalidating the cache:
- Time-based (for all data): You can set a revalidation period for all cached data with the following code.
export const revalidate = <time>
- Time-based (for single request): Set revalidation for a specific fetch request
fetch('...', { next: { revalidate: <time> } });
- On-demand: You can manually revalidate the cache with:
revalidatePath('...');
revalidateTag('...');
- Opt-out Options: You may choose to bypass revalidation
- Whole page:
export const revalidate = 0;
export const dynamic = 'force-dynamic';
- Single request:
fetch('...', { cache: 'no-store' });
- Server Component:
noStore();
3. Full Route Cache
- Where: Server
- What: Caches entire static pages, which includes the HTML and the React Server Components payload. This is advantageous for serving pre-rendered pages quickly to users.
- Duration: The cache persists until the Data Cache is invalidated or a new deployment occurs. This means that your pages will load quickly without needing to re-fetch data as long as they remain valid.
- Revalidation: This cache will be revalidated when the Data Cache is revalidated. Therefore, any changes to the underlying data will automatically reflect when the cache is refreshed.
dynamic = 'force-dynamic';
4. Router Cache
- Where: Client
- What: Caches pre-fetched and visited pages in the user's browser. This improves user experience by reducing load times for pages the user has already visited.
- Duration:
- Dynamic content: Cached for 30 seconds
- Static content: Cached for 5 minutes per session
- Revalidation: You can trigger revalidation of the Router Cache by:
- Using revalidatePath or revalidateTag in Server Actions.
- Calling router.refresh to refresh the current route.
- Modifying cookies with cookies.set or cookies.delete in Server Actions.
- Opt-out: There is no option to opt-out of Router Cache, as bypassing this may lead to issues with user experience.
Conclusion
Understanding these caching mechanisms in Next.js allows you to optimize your application's performance and manage data efficiently. Implementing them effectively will enhance user experience and reduce loading times. Use these strategies to tailor your application's data handling and caching according to your specific needs.
By applying the right caching techniques, you can ensure that your Next.js application remains fast and responsive.